Food Delivery App 10 - Styling Angular Web App with Bootsrap
Adding Bootstrap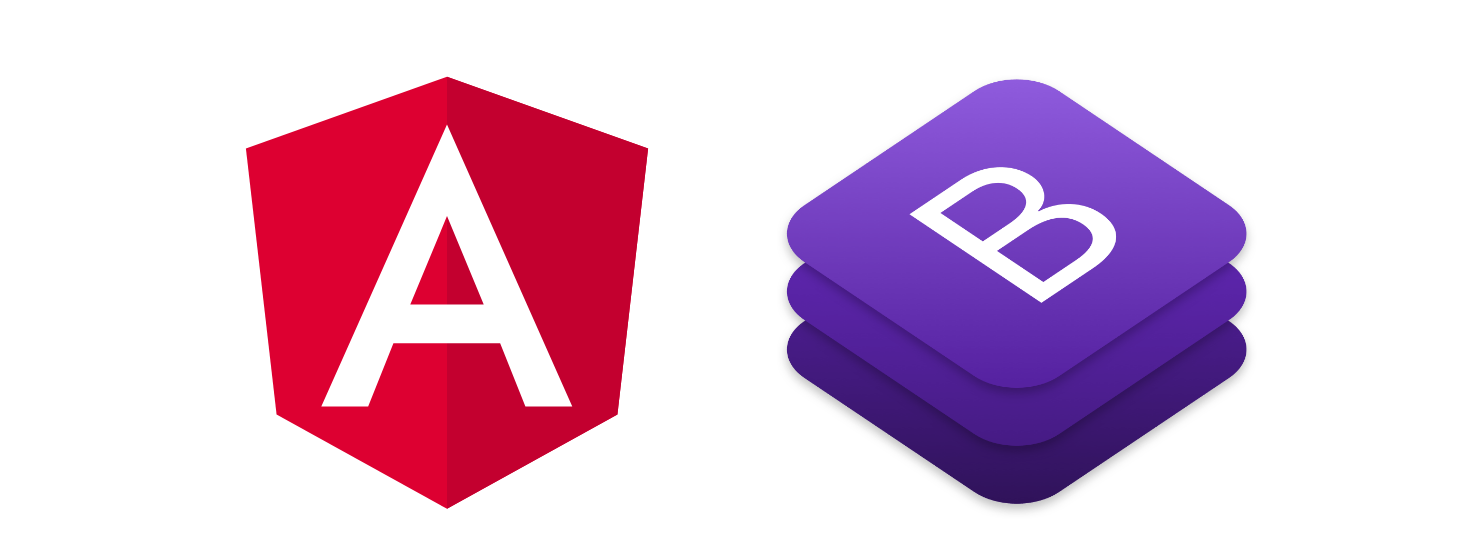
After creating the project, you need to install Bootstrap 4 and integrate it with your Angular project.
First, navigate inside your project’s root folder:
Next, install Bootstrap 4 and jQuery from npm:
(In this case, bootstrap v4.2.1 and jquery v3.3.1 are installed.)
Finally, open the angular.json
file and add the file paths of Bootstrap CSS and JS files as well as jQuery to the styles
and scripts
arrays under the build
target:
Adding A Data Service
After creating a project and adding Bootstrap 4, we’ll create an Angular service that will be used to provide some demo data to display in our application.
In your terminal, run the following command to generate a service:
This will create two src/app/data.service.spec.ts
and src/app/data.service.ts
files.
Open src/app/data.service.ts
and replace its contents with the following:
We add a contacts
array with some demo contacts, a getContacts()
method which returns the contacts and a createContact()
which append a new contact to the contacts
array.
Adding Components
After creating the data service, next we need to create some components for our application. In your terminal, run:
Next, we’ll add these components to the routing module to enable navigation in our application. Open the src/app/app-routing.module.ts
file and replace its contents with the following:
We use the redirectTo
property of the router’s path to redirect users to the home page when they visit our application.
Adding Header And Footer Components
Next, let’s create the header and footer components:
Open the src/app/header/header.component.html
file and add the following code:
A navigation bar will be created with Bootstrap 4, and we’ll use the routerLink
directive to link to different components.
Use the .navbar
, .navbar-expand{-sm|-md|-lg|-xl}
and .navbar-dark
classes to create Bootstrap navigation bars. (For more information about nav bars, check out Bootstrap’s documentation on “Navbar”.
Comments
Post a Comment